Fizz Buzz in CSS
Ever heard of FizzBuzz? To developers, it’s a controversial and oft-used problem to give when applying for positions, but to children it’s instead a game to teach them about division and multiplication.
Briefly, the idea is that you count up in 1s starting at 1 and every time you are supposed to say a number divisible by 3 (such as 3, 6, 9…), instead, you say “Fizz”. If it’s divisible by 5 (that’s 5, 10, 15…), you say “buzz” and if both 3 and 5 divide into it exactly (e.g. 15, 30…), you say “FizzBuzz”.
For developers, there isn’t anything peculiar about this task: it simply uses loops/if conditions but that aside, I’d never seen this before:
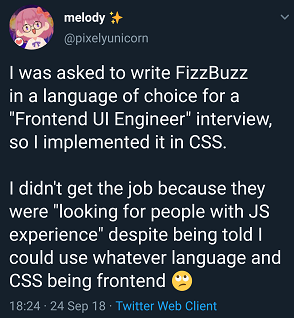
Wow! That got me wondering…how might it be done? This brief article is going to show you one such implementation. I am sure there are more so do get in touch if you know a better way using standard css.
Let’s start with a blank HTML document; we’ll use this to display our numbers.
1 | <!DOCTYPE html> |
Now add some numbers in:
1 | <body> |
In the browser, all is looking mighty fine.

But what about the rules? Well, we’re going to lean on css pseudo-elements.
A CSS pseudo-element is a keyword added to a selector that lets you style a specific part of the selected element(s). For example, ::first-line can be used to change the font of the first line of a paragraph.
The first one we’re going to use here is named :nth-child which matches against elements based on their position amongst siblings. In plain speak, it lets you match against things which are alongside one another at the same level such as…you guessed it: <li>
elements.
One way to use it is to specify which exact element you want, like the 5th. For that, we would combine all three things (the element), the nth-child and the position, like this:
1 | li:nth-child(5) |
The problem is, we don’t just want one element - we want to match against every 3rd or 5th element. With a little tweak, we can do just that using the n
notation. Here’s how it looks.
1 | li:nth-child(3n) |
What that means is we want to match against every 3rd or 5th <li>
element. Let’s see that in action but turning those elements red and blue, respectively, just for this example. Go to the top of your HTML document, and in the HEAD section, add this chunk of CSS:
1 | <style> |
Here’s a screenshot showing that.

Moving closer, right? But, we still don’t see any “fizz” or “buzz”. To fix that, we need to incorporate another pseudo-element: :before.
1 | <style> |
Here, what we’re telling CSS is that for each third line, place the text “fizz” in front of what is there. Equally, for every fifth line, let’s add the word “buzz”. Here’s the effect of that on our text.
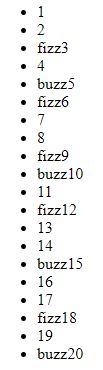
OK…progress. But look what happened at number 15: we lost the “fizz” and overwrote it with a “buzz”. To fix this, we can alter the pseudo-element to use :after; that way, our “fizz” will stay in front of the text for that <li>
and “buzz” will buzz to the end. Here’s the new CSS and the result.
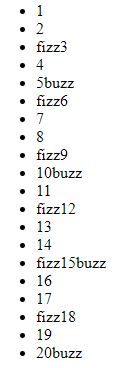
Much better, but how do we get rid of those numbers - we don’t need those when we are using “fizz” or “buzz” or “fizz buzz”. The trick here is to wrap the numbers in an element (a <span>
, in this case) and then just hide it in a puff of smoke. Head back to the HTML and alter the list of numbers to look like this:
1 | <ul> |
The last thing to do is to add in some CSS to remove the <span>
element for any that match our pattern of 3rd or 5th. I’ve provided the whole CSS below and added in those colours from before back in, to make it stand out more:
1 | <style> |
And how does that look in shiny Chrome?
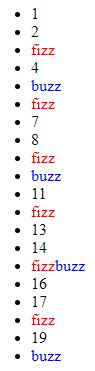
That’s it then - one CSS solution to Fizz Buzz.
If you like, as an exercise, you can add in some JavaScript to dynamically create the list of numbers. If you need a hint, take a look at these JavaScript functions:
Hi! Did you find this useful or interesting? I have an email list coming soon, but in the meantime, if you ready anything you fancy chatting about, I would love to hear from you. You can contact me here or at stephen ‘at’ logicalmoon.com