Removing One Item From an Array in JavaScript
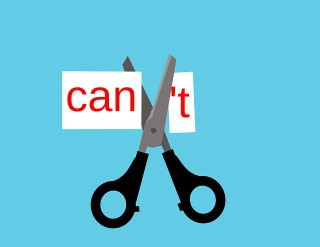
Here’s a tiny tip for you if you find yourself wanting to remove an item from an array that matches a value.
For this example, my array is going to be full of integers, but you can adapt this for other types just as easily.
Let’s begin with an array.
1 | arr = [1, 2, 3]; |
For the purposes of this example, let’s say we want to remove the item 2
which is at position 1
in the array (remember: arrays are zero indexed, which means positions start at 0).
To iterate over this array, we could use a for loop like so:
1 | for (var i = 0; i < arr.length; i++) |
OK, that touches all the elements but what about searching for a specific item to remove? Let’s wrap it in a function to begin with.
1 | function removeItem(arr, item) |
That’s better - we can specify our array, arr
and the item that we are looking for, item
. Time for an if
.
1 | function removeItem(arr, item) |
Almost there! We have zipped through our array and matched the one we are looking for, but what about taking it out? For that, we are going to use splice
(see the docs here). I’ll show you the final code first, and then explain it afterwards.
1 | arr = [1, 2, 3]; |
Splice can do a lot of things such as inserting elements, replacing elements, and of course, removing them, which is what we are doing here.
Specifically, the way we’re using it is to first find the element that we want to remove (line 5) and when found, i
will point to the index position that that element resides. Using splice
we tell JavaScript to remove 1 element (second parameter) from that location, in-place. The in-place portion is important. Often, functions return copies of arrays but in this case, JavaScript alters the array itself, so we don’t need to re-assign any return value from it.
Copy the code out, remove some items, and try and have a hell of a time :-)
Hi! Did you find this useful or interesting? I have an email list coming soon, but in the meantime, if you ready anything you fancy chatting about, I would love to hear from you. You can contact me here or at stephen ‘at’ logicalmoon.com