Creating a Tip Box in CSS/HTML5
I’ve recently been reading the excellent Professional Javascript for Web Developers by Nicholas Zakas (@slicknet) and couldn’t help but admire the way tips are shown in the book.

That got me thinking…how would I make something like that for my blog using CSS and HTML5? Let’s break down the elements of that image into pieces and see how we could implement the various parts.
- In the tip-box (as I will call it) there is an image, text and an area which holds these elements.
- It has a double lined border around the edge.
- It has a (light) coloured background.
- The text is in italics.
- The box is indented on the left and right of the page, offsetting it.
- There seems to be a blank border around the text and,
- There is an image of a pencil, overlapping the left side of the border.
To solve this, we need to create the elements in HTML and then format them using CSS.
1. The Elements
For this, in my HTML, I wrapped everything in an ASIDE element because it is related to the content but as an extra piece of information. Then I added an image which represented the pencil, followed by the text, wrapped in P tags (for the phrasing content – or paragraph). This is what it looks like:
1 | <aside> |
The most obvious thing above is the pencil and that took some contemplation. My options were to draw one or use someone else’s and in that case, of course it needed to be royalty-free. Plus the fact that I am quite picky meant that I spent a while on this. I was lucky enough to see one that I liked, however, (even though it wasn’t quite like the one in the book) at the i2clipart.com website in this one by _Darth Smoo _(Thank you!).
If you choose custom size from the size button, it even lets you enter your own specific pixel dimensions. I chose 50px by 50px. This constitutes the actual information. Next I needed to style it.
2. Adding a Double-Lined Border
Before beginning, I needed to add some identifying information to the tags so that CSS knew which elements I was referring to. That’s pretty easy - just add a class attribute for each of the main elements. It needed to be a class because I wanted ALL tip boxes to look like this and not just one (which is what I would end up with if I used the _id _attribute instead).
1 | <aside class="tip"> |
Notice that I didn’t add one to the P element. I’ll come back to that later. OK, borders. I needed to wrap the whole thing, so that meant the ASIDE tag, but luckily, CSS let’s you specify that easily.
1 | aside.tip { |
You can easily see where I reference the class. In this case, I was only interested in affecting ASIDE tags. There are three attributes in how I used the border property: the fact that I want the line doubled, the amount of space to use (3 pixels) and that I want the non-colour of black. One caveat - make sure that the width of the border is big enough to accommodate the lines. You need a pixel (at least) for each line and one for the gap. I had some fun trying to work out why my choice of 1px didn’t show up (sigh :-) ) I meant I wanted the lines to be 1 pixel in width when the parameter is specifying the total space used!
3. The Background Colour
This one is straight-forward. I quite liked having grey and I achieve that here:
1 | aside.tip { |
What this really means is the colour: #ccccdd because as it stands, if you only use three hexadecimal characters, it repeats each one as a kind of short-hand.
4. The Text in Italics
This is created using a font style as follows:
1 | aside.tip { |
5. Indenting the Tip Box
For this, I am going to use a tip I picked up from the brilliant book: Learning Web Design by Jennifer Robbins (@jenville). I needed to set the ASIDE element to be a certain size and then set the left and right margins to auto. That caused the box to shrink a little and then centre itself.
1 | aside.tip { |
6. and 7. Adding the Image
These two are close cousins so I will explain how I did both, together. Before I begin, I want to credit @scaron and his blog entry on how to handle the arrangement. My attempts left me with a leading indent on the first line of the text that I could not shift, no matter what! His article explains a slightly different effect, but I was able to use his ideas to accomplish what wanted. Step one is to add some padding around the edge of the text. I did that using the padding attribute like so:
1 | aside.tip { |
What this means is that there will be a 25 pixel gap at the top and bottom, with a 50 pixel gap either side. Then onto the image (the pencil).
1 | img.tip { |
Here, I push the image 25 pixels to the left from where it would ordinarily go, and then 10 pixels down. This places my pencil in the middle of the vertical, left-doubled, line (mouthful alert) and a little down, leaving a gap below the horizontal top line. I put the width and height properties in there but in this case, they are redundant because I know my image is 50px by 50px. I just wanted to show you how you would do it for your image, should you choose to. If you have followed along so far, this is what you would see, but it isn’t quite there yet:
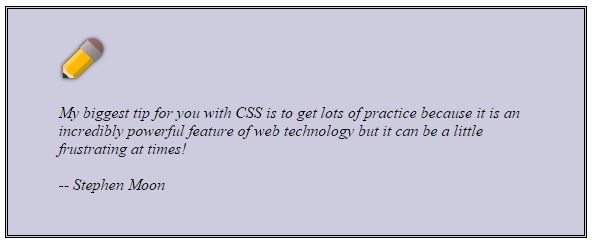
The last step is to set the ASIDE element to use relative positioning and then for the IMG to use absolute.
1 | aside.tip { |
But why? This is where Stéphane Caron’s article comes in. He explained that by doing this, the image doesn’t take account of the padding of the container, allowing it to be placed on top of that space (the padding).
The Final HTML, CSS and Box
Oh. Remember I said I would explain why I didn’t need to set the class for the P tag? Well, that’s because of inheritance. All the important CSS styling that I wanted was passed down to the children of the ASIDE tag, automatically, so I didn’t need to. Cool eh? Lastly, I had a few of my own preferences such as using a sans serif font and justifying the text which you can see below. Hope you like it! Note: the gap at the top is slightly larger but it’s my theme’s settings at work with their dark arts!

My biggest tip for you with CSS is to get lots of practice because it is an incredibly powerful feature of web technology but it can be a little frustrating at times!
1 | aside.tip { |
1 |
|
Hi! Did you find this useful or interesting? I have an email list coming soon, but in the meantime, if you ready anything you fancy chatting about, I would love to hear from you. You can contact me here or at stephen ‘at’ logicalmoon.com