Using Moment.js to See How Old You Are
Sometimes I forget how old I am. Really! When asked my age, I need to take a couple of seconds to think about my age in worry lines, then adjust it to what I am in actual fact. That got me thinking.
I’d heard about moment.js when we were working on a SpiceWorks add-on at work so thought I would take a look to see how easy it is to use. As I found out, it’s quite simple, so here’s a small worked example to show you one use-case for it. To begin, here’s what we are hoping to achieve.
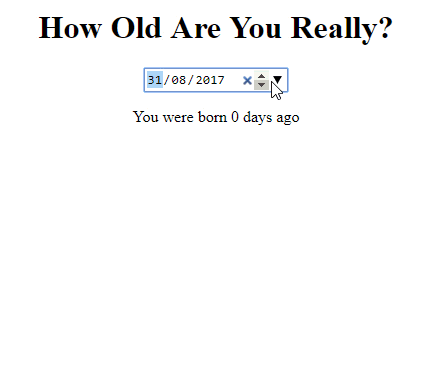
Incidentally, if you have tried adding an animated gif into your Wordpress article and ended up with a static image, try these steps to get around that.
OK, back to me, me, ME.
Let’s start with the basic HTML page. Save this as birthday-picker.html
1 |
|
Now we can add in a reference to moment.js into the head section. You can find that here, but for my project, I downloaded it and added it to the same directory that this HTML resided.
1 | <script src="./moment.js" type="text/javascript"></script> |
Some HTML elements are needed next to handle the input and a title. They are all quite straight-forward and reside in the body section.
1 | <body> |
You can see from the above that I will sneak in the actual results of this into the span but for now, we can assume we will start with the current date, so it will be zero days.
One more thing is to make it look a little prettier so we can embed some CSS for that in the head section. Note, this is only a demonstration - really, this should sit in another referenced file, of course :-)
1 | <style> |
That gives us the basic layout, so next we can do the actual meat of this. We need to make something happen when the user changes the date picker. For that, we need to add an attribute to the input element to make a function call, like so:
1 | <input id="chosen-date" type="date" onchange="calculatePeriod()"> |
But what will be called? At the end of the HTML, add the following script block:
1 | <script type="text/javascript"> |
Let’s break this down a little to understand what is exactly happening.
The first thing we do is to create a moment object using the value in the date picker. Note the format string at the end: “YYYY-MM-DD“. That’s telling the moment constructor what to expect from the value in the date picker, which is namely, a four digit year, hyphen, two digit month, hyphen and you can guess, two digit day of the month.
Once we have that, we then work towards filling in the results within the “output-result“ element. I begin by starting off with the word “begin“ (you will see soon why, when we look at the moment docs). Then, taking the object we created (chosenDate), we call the “from“ method and pass in the current date. So, basically, we are asking moment to tell us what the timespan was between now (new Date) and the date chosen by the picker (chosenDate). The output of that is the English translation of that period of time.
What does that look like in principle? Well, assuming today is the 31st of August (a safe bet), these are the results I get when I plug in various values.
01 Aug 2017 : You were born about a month ago |
Yes, you read that right. For it to say it is about 2 months, you actually have to go back almost a month and a half, and before that, at least from the 1st of this month, its still a month. Here’s why:
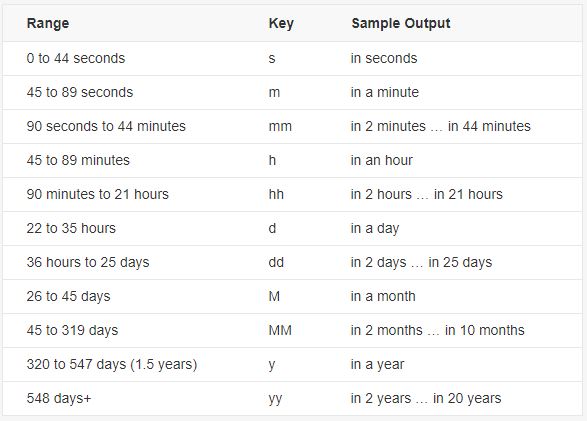
Almost done; one last thing to finish up is to pre-fill the date picker, which is done by adding two more lines above the function.
1 | <script type="text/javascript"> |
Again, as you can see, I’m using moment.js to do some string formatting for me.
There’s lots more this can do and in fact, ways in which you can use existing methods to avoid getting the current date, but I will leave that for you to experiment with.
I’ve added this to one of my webpages here so that you can have a play with it and here are the docs for you to chew on, too.
Hi! Did you find this useful or interesting? I have an email list coming soon, but in the meantime, if you ready anything you fancy chatting about, I would love to hear from you. You can contact me here or at stephen ‘at’ logicalmoon.com