A Small Twitter Project
I was on my way home tonight when I saw this on Twitter.
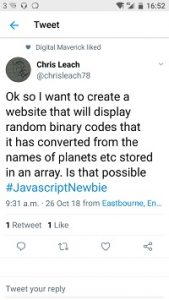
Is it possible, though? Definitely! But what exactly did the tweeter mean by their request? My interpretation is that we need a web page which uses:
- A string array of planets.
- Each planet to be written with capital letters.
- The result to be shown as a series of binary representations of those ASCII capital letters.
- Each binary series to be separated by a space and,
- All binary strings to be left padded with zeroes to a size of 8 characters. That matches 8 bit bytes which is more than enough to store these and makes things consistent.
Additionally, I have decided:
- To keep things simple - no created functions or use of more complex in-built functions like map() or .each() plus,
- To explain it all in some detail.
- In addition, I’ve chosen to use capital letters because I imagine the children using this will have to convert the binary back into letters and uppercase (only) will make that easier. “A” is represented by the number 65, “B” is 66 and so on.
That’s enough chitter chatter - let’s take a look at some basic HTML to present this to the user.
1 |
|
Type the above into a text document and name it “planets.html“. Looking at the code, you can see that we have a suitable title:
1 | <title>Binary Planets</title> |
Then there’s a heading to say what this is all about:
1 | <h1>Binary Planets</h1> |
Next, we have a place to store the results. You can see that I have imaginatively named this element “planet“ but for now, it’s empty. Let’s not forget a link to the JavaScript that we will be writing at the bottom. Without that, this page would be dead in the water.
1 | <script type="text/javascript" src="planets.js"></script> |
Time for the actual JavaScript. Type the following into another text file and name it “planets.js“.
1 | planets = ["NEPTUNE", "EARTH", "PLUTO"]; |
This is all fairly standard JavaScript, but let’s take it line by line.
1 | planets = ["NEPTUNE", "EARTH", "PLUTO"]; |
Our planets are stored in an array and you can easily add new ones on the end by following the same pattern. How do you refer to “NEPTUNE“ though? To do that, you need to understand a couple of things. Firstly, to refer to a position in the array, we use square brackets and an index number. Here’s an example looking at the item at index position 1:
1 | planets[1] |
That’s gives us “_EARTH” _by the way. OK, but what’s all this index number stuff about? Surely EARTH isn’t the first planet in the array? For that, you need to understand arrays begin with element 0 and go up to whatever their length is, minus 1. What would that make _PLUTO_s position, then? Well, there are 3 elements (“NEPTUNE”, “EARTH” and “PLUTO”) and 3 minus 1 is 2, so 2 is the position. OK, great, we’re zipping through this! You’re now ready to tackle the next statement.
1 | chosenPlanet = planets[Math.floor((Math.random() * planets.length))]; |
Math.random() is a handy in-built function that will return a number between 0 and 1.
1 | > Math.random() |
Pretty cool, but not much use for our index position since that needs to be a whole number (integer). Plus, what if we want position 1 or 2? Let’s multiply it by the number of elements:
1 | > Math.random() * planets.length |
Getting better but it would be nice to remove that decimal portion. That’s where the floor() function comes in; it basically rounds downwards. Using that, this becomes:
1 | >Math.floor((Math.random() * planets.length)) |
Brilliant! We have an index number we can use and if we run this several times, we will get a whole range of numbers between 0 and 2, inclusive. The final piece of the puzzle is to refer to that element in the array, so back to the whole statement:
1 | chosenPlanet = planets[ Math.floor( (Math.random() * planets.length) )]; |
As you can see, I placed some spacing in there to make it more obvious. I’m going to take the next few lines as one block, now:
1 | binaryText = ""; |
binaryText is going to store our result and will end up looking a little like the following:
1 | "01010000 01001100 01010101 01010100 01001111" |
To start with though, we place an empty string inside to make sure we begin afresh. How does the final result get there, though? Well, the “for“ loop iterates over our chosen planet’s string, letter by letter. So, if it had chosen “EARTH“, it would run 5 times, going from 0 to 4, inclusively.
1 | i = 0 |
As soon as “i“ became 5, it would no longer be less than the length of the string, “EARTH“ - that test happens in the middle portion (i < chosenPlanet.length). Furthermore, adding one each time occurs at the i++ bit and simply means, take the value of i, add 1, then store the value back into i. In this example, we’re now doing something 5 times, going from 0 to 4, but what exactly do we do each time? That’s where this statement comes in:
1 | binaryText += chosenPlanet\[i\].charCodeAt(0).toString(2).padStart(8, '0') + " "; |
There’s quite a lot here so I am going to get out my sharp knife and chop it up.
1 | binaryText += |
This means, take what’s in binaryText (nothing at first) and add on what comes to the right.
1 | chosenPlanet[i] |
This represents the letter of the planet. When “i“ is 0, it’s “E“ in our example.
1 | charCodeAt(0) |
Taking that letter, return the ASCII code for it. “A_”_ is 65, remember. For “E“, its 65 + 4, so 69.
1 | toString(2) |
Now convert that number into binary. The 2 here represents base 2.
1 | padStart(8, '0') |
Is the resulting string less than 8 characters? If so, pad the left hand-side with “0”s up to a length of 8 characters.
1 | + " " |
Finally, stick a space on the end because that space separates our binary strings. Actually, I did say finally, but have you noticed I didn’t mention something? That’s right, the periods (“.”). That’s a nice feature called a fluent interface and effectively means we can call one function, take the value(s) it returns and pass those onto the next function, rather like a chain. We’re at the final hurdle - hold onto your reins!
1 | document.getElementById("planet").innerHTML = binaryText; |
This small statement goes into the HTML document and finds the element which is labelled: “planet“. Got it? Look back at the HTML code above if you have forgotten what that is. Now set the HTML inside that element to equal our text string. Right at the beginning I told you that it was empty. Well, it still is, but now will be replaced by our binary text. Phew! We’re done. You can take a look at this running here. Keep refreshing the page to see more examples.
Hi! Did you find this useful or interesting? I have an email list coming soon, but in the meantime, if you ready anything you fancy chatting about, I would love to hear from you. You can contact me here or at stephen ‘at’ logicalmoon.com