Understanding Types
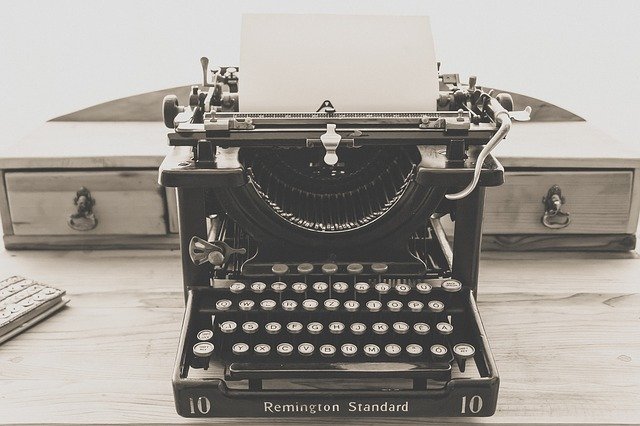
This is a short article giving a quick and dirty introduction to what types are in programming languages. By types, I am referring to the things people talk about when they say so-and-so language is “weakly typed” or “statically typed” - that kind of thing.
To begin with, we need to explain what a type is, since after all, that’s the focus of this article. One way to describe a type is to say it names the kind of “thing” a variable is.
If we were to use an analogy with the real world, we could discuss animals. A dog is a type of animal. So is a cat, or a horse. When you look at these things, you can (usually) recognise them instantly, or at least, I hope so!
So what about programming languages? What types are we talking about, here? Well, how about integer; that’s a type. An integer is a whole number without a fractional part such as 12, -300, or a million. There are also strings which are a series of characters. Of course, there are many more and in some languages, it is even possible to make your own types.
Going back to our analogy, it’s probably worth mentioning where things like unsigned integer, or long come in, as you can find in some programming languages. Taking the first example (unsigned integer) - an integer which can only be positive - it has no sign (other than positive). If we refer to our animals again, I suppose that would be the equivalent of having a dog (the type) but it being a particular breed of dog like Boxer, or Bulldog.
OK, so hopefully we have nailed down what a type is but what about some of those other prefixes like static, dynamic, strong and weak? Let’s take each one in turn.
Static Types
A static type is one where the variable is given a type before you use it. In a language like C#, you (usually - there are always exceptions!) need to declare your type before it can be used. So, if I wanted to have an integer as a variable and assign a value to it, I could do it like this:
1 | int myShinyNewVariable; |
Dynamic Typing
Dynamic types don’t need to be declared (or specified), before you use them. That means I can create a variable and the interpreter will do some custom voodoo to work out which type it should be without me explicitly telling it. PHP is like that and that is why you will see things like this littered through the source files:
1 | $myShinyNewVariable = 1000; |
Here, I’ve created a new variable named myShinyNewVariable
and immediately assigned the value 1000 to it. PHP, in it’s wisdom, decides thats an integer type and creates a place in memory for it. That covers static and dynamic but how about the other two:
Strong Types
What do you think of when you see that phrase: strong type? Something beefy like a double or a long unsigned integer? Well, here, what we’re talking about are types that, once they have been decided, can’t be changed. Java is an example of a language which is like that which you can look at in this example:
1 | boolean hasDataType; |
Now that hasDataType
has been marked as a boolean, it will always be a boolean, no matter how hard it tries to change it!
Weak Types (or Loose Types)
Weak types (sometimes known as loose types) on the other hand are the types that can change from one to another. So, as an example, an integer variable with one value, could later become a string, with another. The name of the variable won’t change, but its type, could. Using PHP again, you might do something like this:
1 | $salary = 1000; |
salary
switches from being an integer into a string, just like that!
Hopefully you found that useful and in some way it clears up a bit of confusion on types.
Till the next one…
Hi! Did you find this useful or interesting? I have an email list coming soon, but in the meantime, if you ready anything you fancy chatting about, I would love to hear from you. You can contact me here or at stephen ‘at’ logicalmoon.com