Fixing Short Echo Tags With CS Fixer
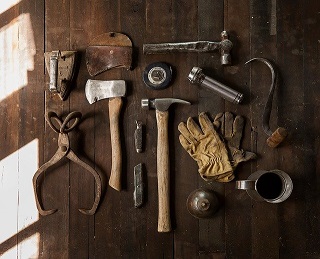
I’m currently working on a legacy PHP application which has a variety of partial HTML files using this style of code:
1 | echo 'hello';> |
I think it looks nicer using the short code version, but only for simple statements like the above. Given a choice, I would rather it looked like this:
1 | 'hello';> |
Changing it manually is a bit fiddly and error prone, as you can imagine. Moreover, I was rather surprised that PHPStorm doesn’t already cater for this with it’s reformatting rules which I wrote about in a previous blog post.
There is a solution though: CS Fixer, and this brief blog post will show you how to install it in PHPStorm and automatically correct those kinds of code styles. Once installed, you can of course get it to do much, much more.
Installing the Binary
There are only a few short steps but let’s start with where we’re going to install the fixer. Begin by changing directory to your project root folder. For this, let’s assume mine is: c:\temp\project
.
Now use composer to install CS Fixer:
1 | $ composer require --working-dir=c:/temp/project friendsofphp/php-cs-fixer |
I’ve chopped the above output a little for brevity at the three elipsis, of course. Running this will produce much more logging.
Adding a Configuration File
We’ve got the fixer installed but need to now tell it how to handle those short tags. For this, create a file named: .php-cs-fixer.dist.php
in the project’s root folder. This configuration was based on the one from the original repo and should be placed inside it:
1 | <?php |
There’s a lot of information there, but for now, focus your attention on this part:
1 | $config |
Inside the rule array, we’ve added one which CS Fixer will recognise related to short tags (echo_tag_syntax
). You can find out the full options on this page, but basically, we are choosing to replace the long form tags with the short, and only in cases where we are simply echoing items.
Configuring PHPStorm to use CS Fixer
Everything is ready to use - we just need to tell PHPStorm all about it, which is what we’ll do now.
- Go to settings via
File > Settings...
orControl-Alt-S
- Click on
Tools > External Tools
- Click on the
+
icon at the top-left of the panel on the right. The following screen (albeit unfilled) will appear:
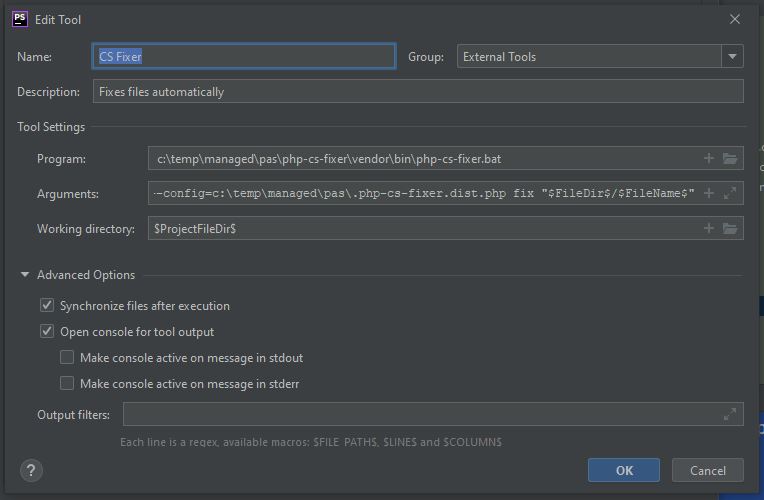
Now set the following fields as shown:
Name
>CS Fixer
Description
>Fixes files automatically
– not essentialProgram
> Path to the CS Fixer program. For me, that’s:c:\temp\project\vendor\bin\php-cs-fixer.bat
Arguments
>--verbose --config=c:\temp\project\.php-cs-fixer.dist.php fix "$FileDir$/$FileName$"
Working Directory
>$ProjectFileDir$
- Finally click
OK
Adding a Key Binding
We’re almost done. To make things a little easier, let’s bind some keys to run CS Fixer automatically. In PHPStorm, do the following:
Control-Alt-S
orFile > Settings...
- Click on
Keymap > External Tools > External Tools > CS Fixer
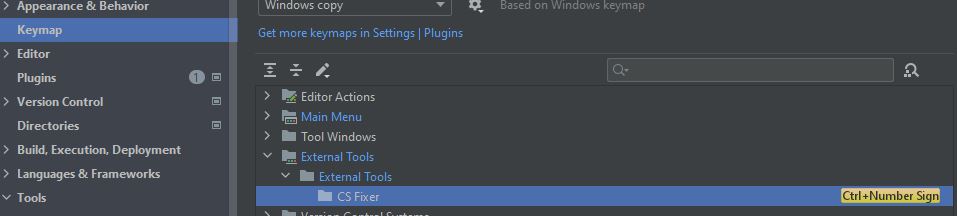
- Press Enter (or click the pencil icon above)
- Click
Add Keyboard Shortcut
- Press your key combination you would like to use:
- I chose
Control-#
- PHPStorm will warn you if you choose one that is already assigned, though, so press away
- I chose
- Click OK.
Testing CS Fixer
Open up a PHP file which uses the long tag echo format, and press your key combo. An output window will pop-up, followed by a lot of verbose output and then CS Fixer will hopefully do its magic!
Here’s some example output when I ran it last.
1 | c:\temp\project\vendor\bin\php-cs-fixer.bat --verbose --config=c:\temp\project\.php-cs-fixer.dist.php fix C:\temp\project\partial.phtml |
Final Words
In this example, I installed CS Fixer into the project folder, but you might choose to install it somewhere centrally for all projects. Just adapt the commands above when you run composer
and remember to point the configuration file parameter to somewhere central, too.
Hi! Did you find this useful or interesting? I have an email list coming soon, but in the meantime, if you ready anything you fancy chatting about, I would love to hear from you. You can contact me here or at stephen ‘at’ logicalmoon.com