Using Get-ChildItem Results in PowerShell
A colleague had a problem using Get-ChildItem in PowerShell.
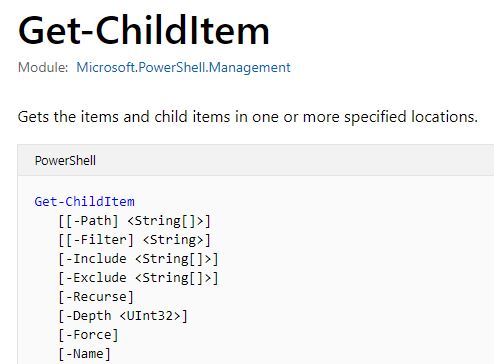
It would return results just fine but there was some confusion over what exactly was being returned. The issue arose because sometimes, when you did this:
1 | $files = Get-Child-Item -Filter *.csv -Path C:\temp |
it would return a number and sometimes it wouldn’t, coinciding with whether there were none, one or more files. Can you guess why?
To understand what is going on, you need to realise that what this commandlet returns isn’t always going to be an array of file objects; sometimes, it is just one single object or even null. We can see this in action next. In C:\ytemp, ensure there are no CSV files and then run this.
1 | $filesFound = Get-ChildItem -Filter *.csv -Path C:\temp |
Now, add one file, followed by one more, each time, running the above script. You should see each showing the correct number. What if we wanted to print out the file details? For that, we just need to extend it a little.
1 | function PrintFileDetails ($fileDetails) |
So, to recap, Get-ChildItem returns:
- Null if there are no items at all.
- A single file object for one object/file and,
- An array for 2 or more found objects.
Hope that helps in excess of 0.0000003% of the world :-)
Hi! Did you find this useful or interesting? I have an email list coming soon, but in the meantime, if you ready anything you fancy chatting about, I would love to hear from you. You can contact me here or at stephen ‘at’ logicalmoon.com