Creating an Imgur Snapchat-Like Screen Capture Tool in Python
A friend wrote a tool which can take screen shots of his PC and upload them to Imgur. There are quite a few programs like this and it’s pretty useful when sharing images, but he has another script which can, after a short period of time, automatically delete the image from Imgur.
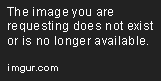
Why would you want to do this? Aside from the many uses Snapchat is put to, I can think of some circumstances in which this might be useful.
For example, let’s say that you only need to share the image temporarily or perhaps the image is sensitive (a screen-shot of a new unseen application you are working on) and you don’t want it to (easily) spread around the internet. I’m sure you can think of other purposes. If I had such a tool, what would my requirements be?
- It would need to be able to take a screenshot within a user-define area of the screen. Whole screen captures are waaaaaay to permissive!
- It should automatically upload the image to Imgur.
- The link (for the newly uploaded) file should be in my clipboard – I don’t want an additional step of selecting and copying any links. The bonus of that is that it will allow me to immediately paste it into a chat window, for instance. And lastly,
- The image should be automatically deleted after n seconds, the delay for which I can choose.
Implementation Constraints
There are a number of must-haves for this:
- Ideally, I’d like this to be implemented quickly - it would be a nice tool, but I don’t need it that much.
- I’d like to use Python. I’ve used it before and was really impressed with the things it can do, so a little more experience with it could be useful.
- It would be helpful to re-use as many existing libraries and tools as I can a) to assist with the first point, but b) to expose me to other existing functionality.
Let’s break this down the requirements above to see how we might achieve this.
Taking a Screenshot
For this, I could either write my own program or use an existing one. It goes without saying that using an existing one will be quicker, provided it can do what I want.
A quick look at the snipping tool in Windows shows that whilst you can execute it, you can’t control it via the command line, so that’s out. A short Google later and I found this: MiniCap. This is a free (but donations welcome) tool which has the benefit that I can call it from the command line and instruct it to start a region screen-grab. In addition, I can specify a filename to save to. Perfect! The first thing to do then is to download the portable version and save all the files it contains in C:\PyImgurGrabber\bin
. Please do visit the authors homepage too, and consider donating, though; it’s a great tool.
The Python Implementation
Let’s get some prerequisites out of the way first, because you will need these. Do you already have Python installed? If not, you can download that from here and should install it, ready to use. Next, you will need to install some packages. Type these in as shown:
> pip install pyimgur |
If that doesn’t cause the packages to install (your PATH variable might not be set up properly), make sure you CD into a directory which looks a little like: C:\Python34\Scripts
as that is where the pip installer resides. Bear in mind that if you have a different version of Python installed, it might not be “34“. I’ll explain each package as and when we need them, so don’t worry about what they do yet. We should be all set to start our program now.
Running the Screen Capture Program
Here’s how I implemented the functionality to make the screen capture program execute in Python:
1 | from datetime import datetime |
You can see that I’ve saved all images into C:\PyImgurGrabber
and named them with a template of imgur_YearMonthDay_HourMinuteSecond_.jpg
. As for the options to MiniCap, I tell it I want to capture a region, to exit when it has made the capture and what to name each file that is produced.
Accessing Imgur
What luck! Python has a number of existing libraries that you can use :-) The first I found was provided by Imgur themselves called imgurpython, but the documentation is really poor, so I scrubbed that. Then I came across this: pyimgur. It is so much better (read: easier) and in fact has some examples about uploading the image (anonymously) to Imgur. To use it though, you need to set up an account on Imgur and get hold of a client ID.
Creating an Imgur Account and Getting a Client ID
Creating the account is pretty straight-forward - just go to the homepage (www.imgur.com) and choose sign up at the top right. Once that is done, you need to tell them about your application. Go to this address (https://api.imgur.com/oauth2/addclient) and fill in the fields as follows:
**Application Name**: PyImgurGrabber |
Lastly, enter the captcha code and click submit. On screen should now be your client id
and client secret
- make sure you save both strings somewhere safe. We will be using the client id in a short while.
Uploading to Imgur
This is how I accessed the service and sent it the file I had created:
1 | import pyimgur |
Seriously, that’s it!
Note - it references the JPG_PATH
already defined earlier - to make this easier to digest, I am showing each part separately.
You can also see my client id, but don’t use that, not least because it doesn’t work - I’ve changed it for this article.
Also, if you are using Python version 2.7, remember to remove the round brackets from the print statement - it’s a version 3 feature.
OK. So we have now captured an area of the screen, saved it as a file and uploaded it to Imgur. The next step is to get hold of the URL for the new image and place that in the clipboard.
Saving the URL to the Clipboard
If you haven’t already guessed, Python is pretty amazing! All you need to do to save the URL and make it available to the clipboard is the following:
1 | # place imgur url into clipboard so that we can paste it somewhere |
This was why we installed the pyperclip
package - it does all the hard work for us.
Waiting and then Deleting
We’re almost done now. All that is left is to nap for a short while and then delete the image.
1 | # sleep for 120 seconds |
If you are typing this all in, don’t forget to save the file as PyImgurGrabber.py
and place it in the C:\PyimgurGrabber
folder.
Running PyImgurGrabber
There are a number of ways you can run this application.
On the command line (by running cmd.exe), just type:
pyimgurgrabber.py
Using this method will leave your cmd window open so if you are running on one screen and this is getting in the way, you might want to try option 2.Create a shortcut to it and perhaps place that somewhere. To do this, right click on the desktop, New…shortcut.
Then browse to the .py file and click OK. Then click Next and Finish.
If you now right click on the shortcut, you can go to Properties and then in the Run dropdown, change that to “minimized“ before clicking on OK.
Once run, you should see some cross hairs. Select the region of the screen you are interested in and then release the left mouse button. It should then grab it, upload it and make the URL available for you to paste elsewhere. Be quick though, before it gets deleted!
The Whole Script
1 | from datetime import datetime |
You can find this whole script and files on my GitHub page. Hope you find it useful!
Hi! Did you find this useful or interesting? I have an email list coming soon, but in the meantime, if you ready anything you fancy chatting about, I would love to hear from you. You can contact me here or at stephen ‘at’ logicalmoon.com