Setting Classes Based on the Route in Laravel
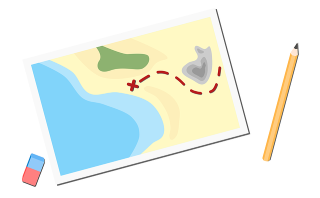
Imagine this situation: you have a blade file that has some navigational buttons, each of which point to a separate page on your site. As you click on each page, you want to use CSS to show which page (button) is active. This is pretty common, of course, and you can see lots of examples of it all over the web. In my case, for my simple demo, I wanted something like this:

I’m using the framework Materialize for this and haven’t chosen highly contrasting colours, but hopefully you get the idea. In this example, the button Courses
has been highlighted because that’s the navigational page that I am on. But how do you do this?
As ever, there are many ways, but the method I am going to show you is pretty straight-forward. All you need to know about the CSS is that when an element in the navbar is marked as active
, its colour changes like that.
Let’s jump to the blade file where you can see how I did it.
1 | <li class="{{ (Request::is('courses') ? 'active' : '') }}"> |
You can see in line 2 that I have an anchor tag which points to a named route called courses
. In terms of the URL, that would be something like: https://example.com/courses
.
In Materialize, though, we represent navbar items using <li>
tags so that’s the part we need to zoom in on when setting the active
class.
The key part, as you can see, is the Request::is('courses')
test that we are making using the ternary operator. If the returned value of that method matches courses
then the test is true, and we return active
. Otherwise, it’s that empty string.
So this URL: https://example.com/courses
would result in this HTML:
1 | <li class="active"> |
and the other navbar items won’t:
1 | <li class> |
What about the root of the URL. E.g. https://example.com/
? Well, we can catch that with a slightly altered test.
1 | <li class="{{ (Request::is('/') ? 'active' : '') }}"> |
If you want to dig a little deeper, look at the official documentation and also, how Str:::is(...)
works since this can also use pattern matching.
Give it a go and let me know if you run into any difficulties.
Hi! Did you find this useful or interesting? I have an email list coming soon, but in the meantime, if you ready anything you fancy chatting about, I would love to hear from you. You can contact me here or at stephen ‘at’ logicalmoon.com